Getting it done with JAVA
Last modified: 16 Sep, 2019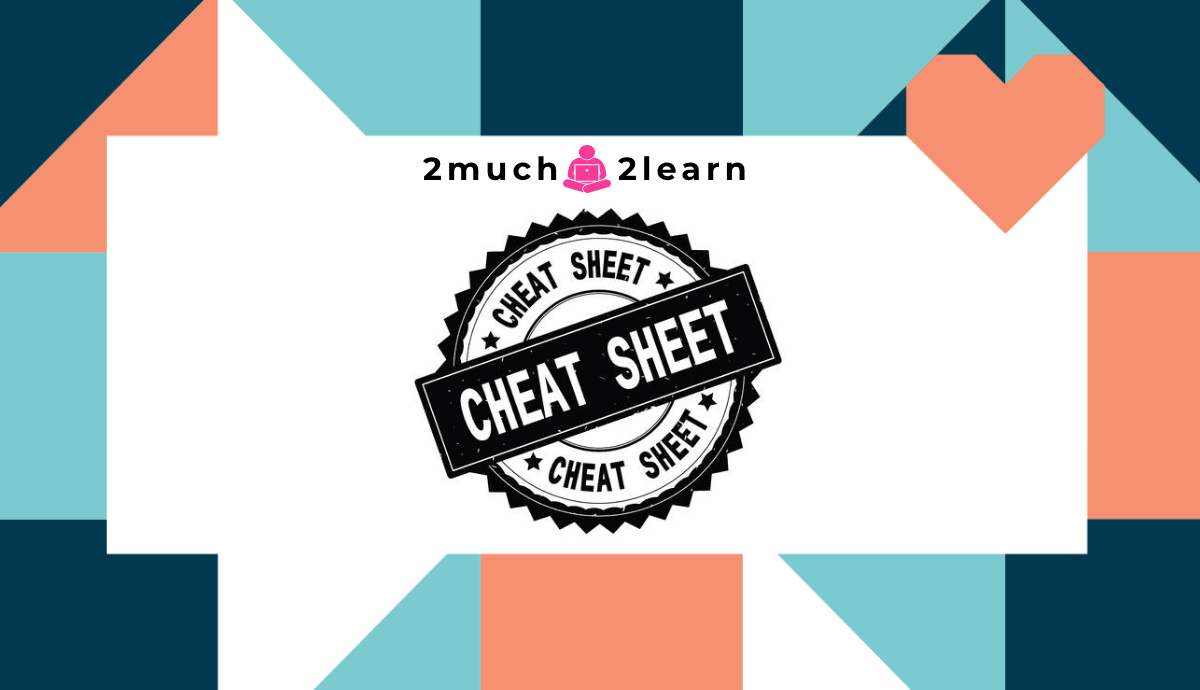
Maven command to create new project from Quickstart Archetype
$ mvn archetype:generate \
-DgroupId=com.toomuch2learn \
-DartifactId=example \
-DarchetypeArtifactId=maven-archetype-quickstart -DarchetypeVersion=1.4 -DinteractiveMode=false
Maven copy all dependencies bundled in war to target folder
$ mvn install dependency:copy-dependencies
Loop n times
// Loop through closed range
IntStream.rangeClosed(1, 8)
.forEach(System.out::println);
// Do some operation
IntStream.rangeClosed(1, 8)
.map(i -> 2 * i - 1)
.forEach(System.out::println);
// build a custom iteration and limit the size of the iteration
IntStream.iterate(1, i -> i + 2)
.limit(8)
.forEach(System.out::println);
BigDecimal
Generating random BigDecimal value from given range
class BigDecRand {
public static void main(String[] args) {
String range = args[0];
BigDecimal max = new BigDecimal(range + ".0");
BigDecimal randFromDouble = new BigDecimal(Math.random());
BigDecimal actualRandomDec = randFromDouble.divide(max,BigDecimal.ROUND_DOWN);
BigInteger actualRandom = actualRandomDec.toBigInteger();
}
}